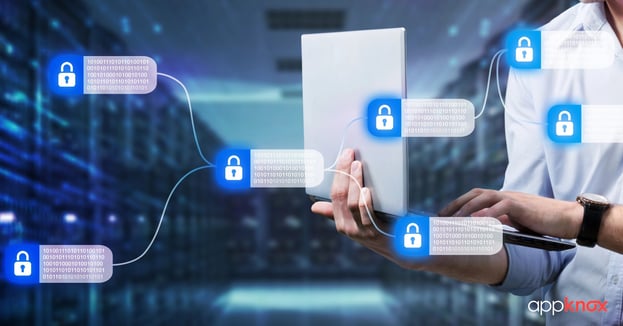
BLOG
BLOG
For this blog post, we are going to work on a pwnable challenge from the HTB cyber apocalypse 2022 CTF. The challenge contains a 64 bit ELF binary and libc shared object.
Challenge description: We got access to the Admin Panel! The last part of the mission is to change the target location of the missiles. We can probably target Draeger's HQ or some other Golden Fang's spaceships. Draeger's HQ might be out of the scope for now, but we can certainly cause significant damage to his army.
Challenge files can be found here.
Let’s start by checking the protections for the binary. I will be using the checksec utility for this.
So, we can confirm that the application has Full Reloc, PIE and NX enabled.
The No eXecute or the NX bit (also known as Data Execution Prevention or DEP) marks certain areas of the program as not executable, meaning that stored input or data cannot be executed as code. This is significant because it prevents attackers from being able to jump to custom shellcode that they've stored on the stack or in a global variable.
Address Space Layout Randomization (or ASLR) is the randomization of the place in memory where the program, shared libraries, the stack, and the heap are. This can make it harder for an attacker to exploit a service, as knowledge about where the stack, heap, or libc can't be re-used between program launches. This is a partially effective way of preventing an attacker from jumping to, for example, libc without a leak.
Playing around with the input, it looks like the application is vulnerable to stack overflow.
We can confirm this by analyzing the application using a disassembler. By analyzing the missile_launcher function, we can see that the function is an allocation stack of 0x50 bytes, but the read function is reading 0x84 bytes from stdin.
Using gdb, we can calculate the offset at which the return pointer gets overwritten. For this, we will be generating a cyclic pattern using the `pattern create` command, and then, on segmentation fault, we will calculate the offset of bytes in the RSP register.
So now we can control the return pointer.
Previously, we had seen that the binary had PIE enabled.
PIE stands for Position Independent Executable, which means that every time you run the file, it gets loaded into a different memory address. This means you cannot hardcode values such as function addresses and gadget locations without finding out where they are. But this does not mean it’s impossible to exploit.
PIE executables are based on relative rather than absolute addresses, meaning that while the locations in memory are fairly random, the offsets between different parts of the binary remain constant.
For example, if you know that the function main is located 0x121 bytes in memory after the base address of the binary, and you somehow find the location of main, you can simply subtract 0x121 from this to get the base address and from the addresses of everything else.
So, all we need to do is find a single address, and PIE is bypassed.
Just as we can leak canary from the stack, we can use a format string vulnerability or any other way to read the address of the stack. This address always needs to be at a static offset from the base of the binary, thus enabling us to bypass PIE completely.
Let’s analyze the binary again for memory leaks or format string vulnerabilities.
This does look like a memory leak. We can use pwntools to confirm this.
from pwn import * |
As seen in the above screenshot, we are able to leak the partial base address of the stack. The last two bytes are still unknown.
Due to the way PIE randomization works, the base address of a PIE executable will always end in the hexadecimal character 000.
5 |
5 |
e |
1 |
2 |
d |
7 |
8 |
? |
0 |
0 |
0 |
So now we are able to leak the first 8 bits of the address, and we know that the last three bits are 000. This leaves us with one missing bit. We can assume the value of this bit and run our exploit numerous times, assuming that, at least in one instance, we get the correct base address.
base = u64(leak[:-1].ljust(8,b"\x00")) #leaking 4 bytes of base_addr from stdout |
Next up, we will be using ret2puts for bypassing ASLR. First, the PLT address of puts() will be needed with the corresponding GOT address to be leaked. Since this is an x64 architecture, it will need an ROP gadget to set up the GOT address of puts in the RDI register so puts could use it ().
The below code snippet will leak the address from libc.
from pwn import * |
Since we are brute forcing one bit of the address as mentioned above, we need to run the exploit in a for loop.
Now, we need to calculate the libc base address by subtracting the puts offset from the leaked address.
After that, we need to calculate the address of the system, exit, and “/bin/sh” string inside libc by adding the respective offsets to libc base address.
The final exploit will be as follows:
from pwn import * |
Again, we will need to run the exploit in a loop.
for i in {1..10};do python3 exploit.py 2> /dev/null | grep 'puts address inside libc' -B 10 -A 10; done |
Happy hacking!!